Day 3: Setting Up Your Environment
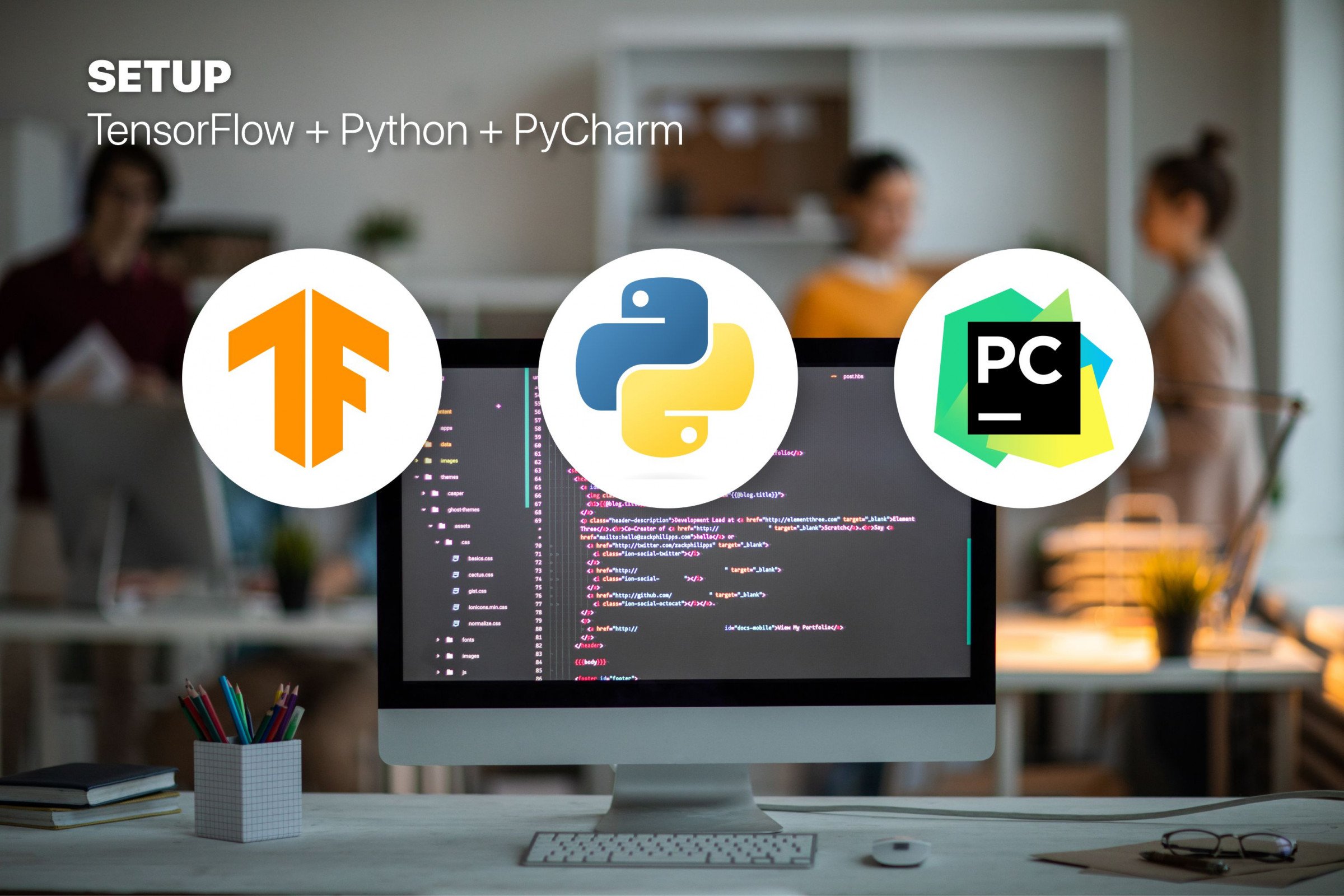
Installing Python and TensorFlow 2.0
Installing Python and TensorFlow 2.0 can be done in a few simple steps.
- First, you will need to download and install Python on your computer. You can download the latest version of Python from the official website at python.org (https://www.python.org/downloads/) . Make sure to download the version that is compatible with your operating system. I recommend 3.7! Most libraries do not compatible with the latest version (Itβs now 3.11). Also, you can have multiple Python version in the same machine by PyCharm (IDE).
- Once Python is installed, you can use the built-in package manager, pip, to install TensorFlow 2.0. Open a terminal or command prompt and type in the following command: pip install tensorflow . Please visit this website: https://www.tensorflow.org/install .
- Next, you will need to install PyCharm, which is an Integrated Development Environment (IDE) for Python. You can download the community version of PyCharm for free from the JetBrains website. https://www.jetbrains.com/pycharm/download/
- Once PyCharm is installed, you can open it and create a new project. In the project settings, you need to specify the interpreter that you have installed previously.
- To verify that TensorFlow 2.0 is installed correctly and that you are able to import it, you can open a new Python file in PyCharm and type the following code:
Run the code, and it should print the version of TensorFlow that you have installed. If you see any errors or issues, you may need to check your installation or configuration.
PyCharm
By following these steps, you should now have Python and TensorFlow 2.0 installed on your computer, and you should be able to use PyCharm to create and run Python scripts that use TensorFlow. PyCharm is a powerful IDE for Python development, it offers features such as code completion, debugging, and integration with version control systems, making it a popular choice among Python developers.
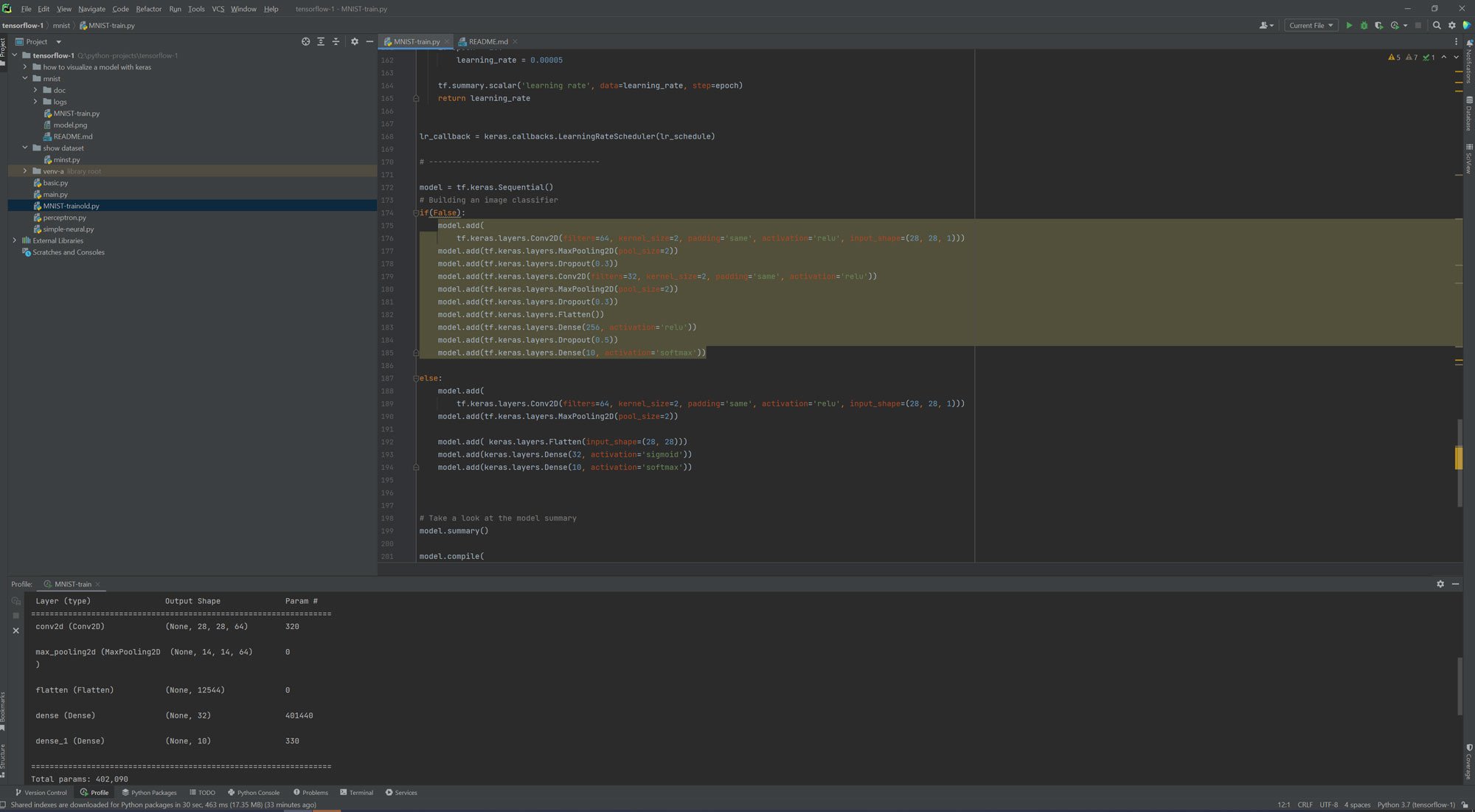
Colab
Google Colab is a free cloud-based platform for machine learning research and development. It allows users to write and execute code in Python and use popular libraries such as TensorFlow and PyTorch without the need for installing them locally. One of the main advantages of Colab is its accessibility. Users can access the platform from any device with an internet connection, making it easy to collaborate with others and work from anywhere. Additionally, Colab provides free access to GPUs and TPUs (Tensor Processing Units), which can significantly speed up the training of machine learning models.
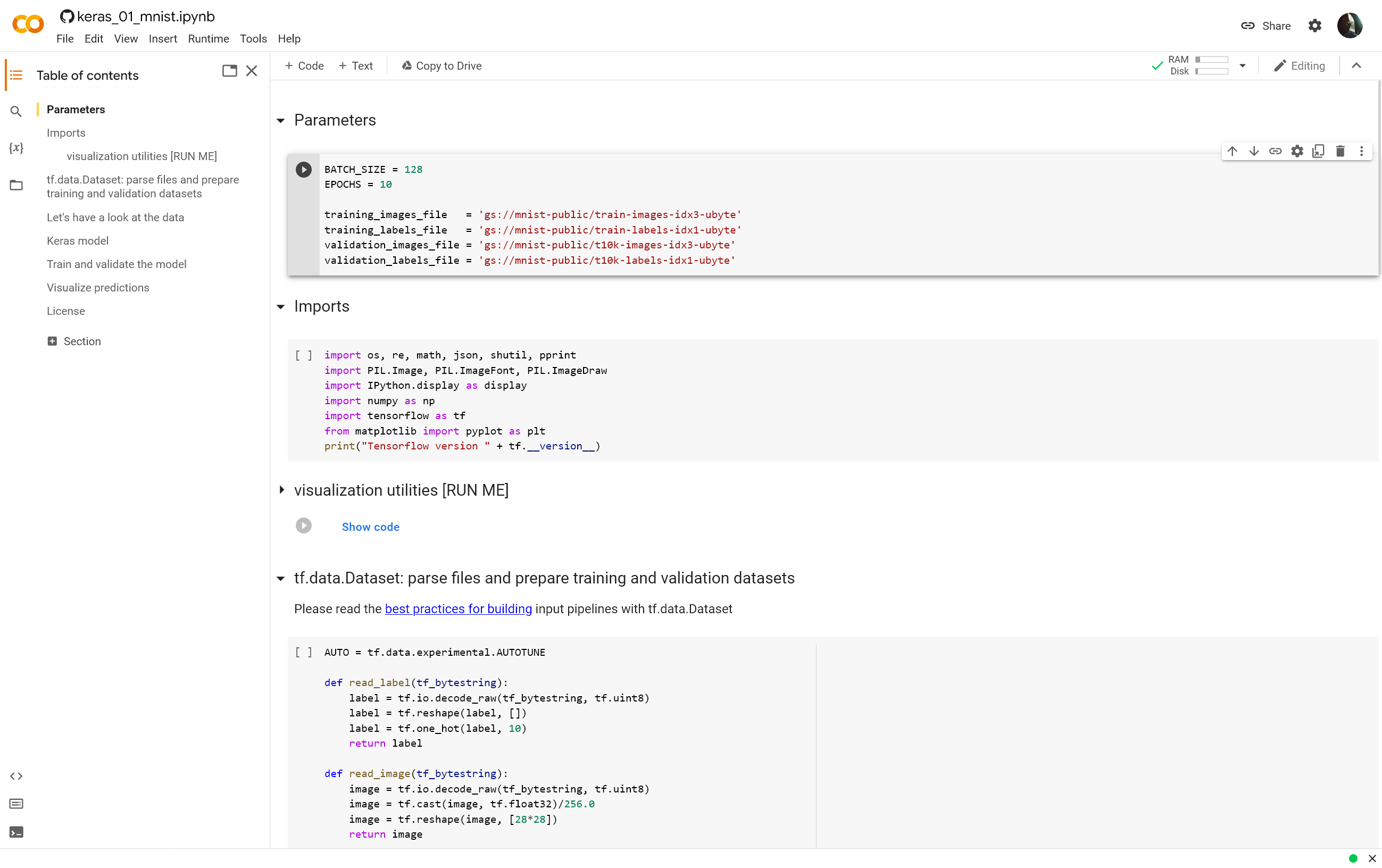
Colab also provides a number of convenient features for working with data, including the ability to easily upload and download files, as well as the ability to connect to Google Drive. This makes it easy to share data and collaborate with others on projects. Colab also offers a Jupyter-like interface which is familiar to many data scientist and researchers. The notebook interface allows users to organize code, documentation, and visualizations in a single document, making it easy to keep track of the different stages of a project.
Another benefit of Colab is that it is integrated with other Google services, such as Google Drive, allowing you to easily access and share files. It also allows you to share your work with others by creating a shareable link. Colab provides a powerful and accessible platform for machine learning research and development, and its free access make it a great option for individuals and teams looking to prototype and explore models without incurring the cost of expensive hardware or cloud resources.
Basics of Python
There are many resources available online to help you learn Python. Here are a few popular options:
- Codecademy: Codecademy is an online learning platform that offers interactive Python courses for beginners and intermediates. The courses include interactive exercises and quizzes to help you practice and solidify your knowledge. https://www.codecademy.com/learn/learn-python
- Coursera: Coursera is an online learning platform that offers Python courses from universities and organizations around the world. The courses vary in length and level of difficulty, and some of them offer a certificate upon completion. https://www.coursera.org/courses?query=python
- edX: edX is another online learning platform that offers Python courses from universities and organizations around the world. The courses cover a wide range of topics and are suitable for both beginners and intermediates. https://www.edx.org/learn/python
- SoloLearn: SoloLearn is a mobile app that offers interactive Python courses for beginners. The app includes quizzes and interactive exercises to help you practice and solidify your knowledge. https://www.sololearn.com/Course/Python/
- Python.org: Python.org is the official website of the Python programming language. It offers extensive documentation and resources for Python, including tutorials and guides for beginners. https://www.python.org/about/gettingstarted/
- Python Software Foundation: Python Software Foundation is a non-profit organization that supports and promotes Python and the Python community. It offers resources, tutorials and events for the Python community. https://www.python.org/psf/
- Python for Everybody: Python for Everybody is a website where you can find a collection of resources for learning Python. It offers tutorials and videos for beginners, as well as a free online book "Python for Everybody". The website is based on the online course from the University of Michigan, which is available on Coursera. https://www.py4e.com/
Basic data types and variables
Python is a high-level programming language that supports several basic data types, including numbers, strings, lists, and dictionaries. Understanding these basic data types and how to work with them is essential for programming in Python. It's important to note that Python is a dynamically typed language, which means that the type of a variable is determined at runtime and not at compile time. This allows for greater flexibility but can also make it more difficult to track variable types and bugs in the code.
- Numbers: Python supports several types of numbers, including integers (e.g. 1, 2, 3), floating-point numbers (e.g. 3.14, 2.718), and complex numbers (e.g. 3 + 4j). Numbers can be used in mathematical operations, such as addition, subtraction, multiplication, and division.
- Strings: A string is a sequence of characters, such as "hello" or "goodbye". Strings are enclosed in single or double quotes, and can be concatenated (combined) using the + operator. Strings also have a number of built-in methods, such as lower() and upper() to change the case of the string and find() and count() to locate andcount substrings within a string.
- Lists: A list is a collection of items, such as ["apple", "banana", "cherry"]. Lists are enclosed in square brackets [] and items are separated by commas. Lists are mutable, meaning that their elements can be changed.
- Dictionaries: A dictionary is a collection of key-value pairs, such as {"name": "John", "age": 30}. Dictionaries are enclosed in curly braces {} and keys and values are separated by colons. Dictionaries are also mutable, meaning that their elements can be changed.
- Variables: In python, a variable is a named location in memory where a value can be stored. Variables are used to store data and are used to reference that data in various parts of a program. The values of a variable can be changed, it's a way of storing and manipulating data.
In python, variables are defined using the assignment operator (=). For example, to define a variable x and assign it the value 7, you would write x = 7. Once a variable is defined, its value can be accessed by referencing the variable name.
Control flow and loops
Control flow and loops are fundamental concepts in programming that allow developers to control the flow of execution of a program and repeat certain actions. In Python, control flow is implemented using control statements, such as if-else statements, and loops, such as for and while loops.
An "if-else" statement allows a program to make decisions based on certain conditions. The basic syntax of an if-else statement in Python is as follows:
A "for" loop allows a program to iterate over a sequence of items, such as a list or a string. The basic syntax of a for loop in Python is as follows:
A "while" loop allows a program to repeatedly execute a block of code as long as a certain condition is true. The basic syntax of a while loop in Python is as follows:
In addition to the above mentioned loops, python also has other types of loops like break, continue and else loops which can be used indifferent situations.
Functions and modules
In Python, functions and modules are two important concepts that are used to organize and structure code. Functions are blocks of code that can be called by other parts of the code. They are defined using the "def" keyword, followed by the function name and a set of parentheses that may contain parameters. Functions can take one or more input arguments and can return one or more values. Here is an example of a simple function in Python that takes two numbers as input and returns their sum:
Modules are Python files that contain functions, classes, and variables. They allow you to organize your code into separate, reusable units and make it easy to share and reuse code between different parts of a program or between different programs. In order to use a module, you need to import it using the "import" keyword. Here is an example of how to import the "math" module and use the "sqrt" function to calculate the square root of a number:
You can also choose to import only specific functions or variables from a module using the "from" keyword, and use them without having to qualify them with the module name.
You can also use the as keyword to give an import a different name
Functions and modules are powerful tools for structuring and organizing your code, making it more readable, maintainable and reusable. They help you to break down a complex problem into smaller, more manageable pieces and make it easier to test and debug your code.
Data Preprocessing and Exploratory Data Analysis
Data preprocessing and exploratory data analysis (EDA) are essential steps in the process of developing artificial intelligence (AI) models using Python. These steps are used to prepare and understand the data that will be used to train and test the AI models. These steps are used to prepare and understand the data that will be used to train and test the AI models.
Importing, cleaning, and manipulating data
Data preprocessing refers to the process of cleaning, transforming, and normalizing the data before it is used to train the AI models. This can include tasks such as removing missing or duplicate data, handling categorical variables, and scaling numerical variables. For example, in python, you can use the Pandas library to remove missing values, convert categorical variables to numerical, and scale numerical variables using the MinMaxScaler function from the Scikit-learn library.
By using libraries such as Pandas, Scikit-learn, Matplotlib, and Seaborn, you can easily perform data preprocessing and EDA tasks in Python and gain insights into the data that will help in building accurate and robust AI models.
Visualizing data to gain insights
Exploratory data analysis (EDA) is the process of analyzing and summarizing the main characteristics of the data, often through visualizations. The goal of EDA is to understand the underlying structure of the data, identify patterns and trends, and gain insights into the relationships between different variables. This can help in identifying any outliers, anomalies or missing values in the data. For example, in python, you can use the Matplotlib and Seaborn libraries to create various types of plots to visualize the data and perform EDA.
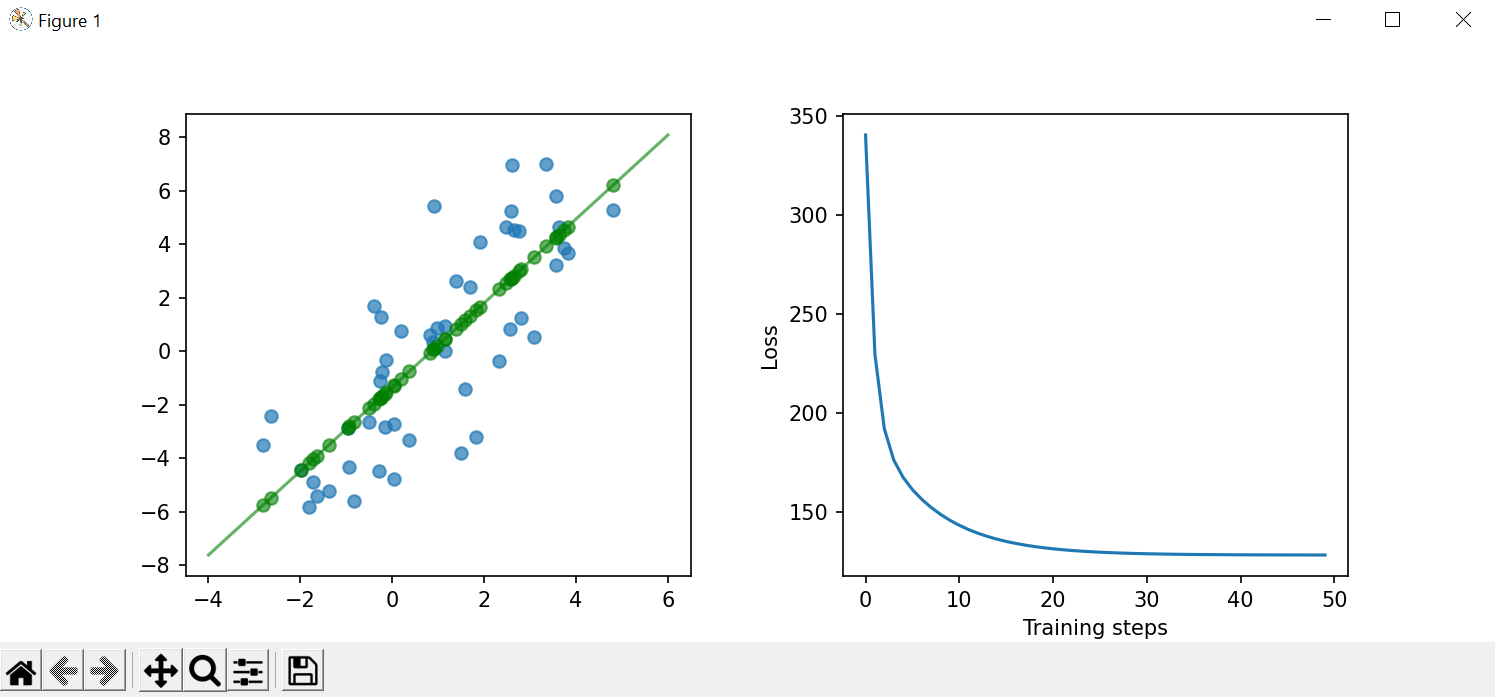
seo.call-to-action.title
seo.call-to-action.money-back
seo.call-to-action.message